KOTLIN
ANDROID
Leveraging Build Configurations for Environment-based Kotlin Application Deployment / Android Studio
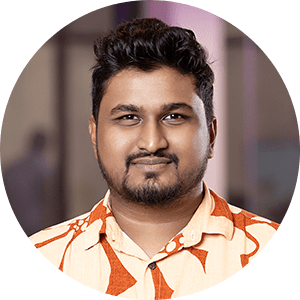
-
Introduction:
In software development, deploying applications across multiple environments — such as development, testing, and production — can present challenges. Managing different configurations, API endpoints, and keys for each environment often requires meticulous attention to detail and can lead to errors if not handled properly. In this article, we explore how to effectively manage environment-specific configurations in Kotlin applications using build configurations.
Understanding Build Configurations:
Build configurations are an integral part of modern application development. They allow developers to define different sets of configurations, resources, and behaviors for various deployment environments. By leveraging build configurations, developers can seamlessly switch between environments during the build process without modifying the codebase.
Setting Up Build Configurations in Kotlin:
In Kotlin applications, build configurations are typically defined in the build script (e.g., build.gradle.kts). Here’s a step-by-step guide to setting up build configurations for a Kotlin application:
- Define Build Flavors:
- Build flavors represent different deployment environments, such as development, testing, and production.
- Each build flavor can have its own set of configurations, including API endpoints, keys, and resource files.
In your build.gradle.kts, define build flavors for different environments:
android {
...
flavorDimensions("environment")
productFlavors {
dev {
dimension("environment")
// Define dev-specific configurations
}
qa {
dimension("environment")
// Define QA-specific configurations
}
prod {
dimension("environment")
// Define production-specific configurations
}
}
}
2. Configure Build Types:
- Within each build flavor, developers can configure different build types, such as debug and release.
- Build types allow for further customization and optimization, such as enabling debug logs or code obfuscation.
Configure different build types within each flavor:
android {
...
buildTypes {
release {
// Release build configurations
}
debug {
// Debug build configurations
}
}
}
3. Define Environment-specific Configurations:
- In the build script, define environment-specific configurations using buildConfigField or resValue functions.
- For example, define server URLs, API keys, and other constants as build configuration fields.
Define environment-specific configurations using buildConfigField:
android {
...
buildTypes {
...
}
productFlavors {
dev {
buildConfigField("String", "SERVER_URL", ""https://dev.example.com"")
}
qa {
buildConfigField("String", "SERVER_URL", ""https://qa.example.com"")
}
prod {
buildConfigField("String", "SERVER_URL", ""https://prod.example.com"")
}
}
}
4. Accessing Build Configurations in Kotlin Code:
- In Kotlin code, access build configurations using the BuildConfig class provided by the build system.
- BuildConfig class exposes the defined build configuration fields as constants that can be accessed at runtime.
In your Kotlin code, access the build configurations using BuildConfig class:
val serverUrl = BuildConfig.SERVER_URL
Implementing Environment-specific Logic:
With build configurations in place, developers can implement environment-specific logic within the application code. For instance, developers can dynamically switch API endpoints, configure logging levels, or enable/disable features based on the current build flavor.
Based on the build flavor, you can implement logic to handle environment-specific behavior:
if (BuildConfig.FLAVOR == "dev") {
// Development environment-specific logic
} else if (BuildConfig.FLAVOR == "qa") {
// QA environment-specific logic
} else if (BuildConfig.FLAVOR == "prod") {
// Production environment-specific logic
}
Automating Environment Switching:
To streamline the development workflow, developers can automate environment switching using continuous integration and deployment (CI/CD) pipelines. By integrating CI/CD tools such as Jenkins, GitLab CI, or GitHub Actions, developers can trigger builds for specific environments based on Git branch names or commit tags.
Utilize CI/CD pipelines to automate environment switching based on Git branches or tags:
workflows:
dev:
name: "Dev Workflow"
...
branch_patterns:
- pattern: 'dev'
include: true
qa:
name: "QA Workflow"
...
branch_patterns:
- pattern: 'qa'
include: true
prod:
name: "Production Workflow"
...
branch_patterns:
- pattern: 'main'
include: true
Best Practices and Considerations:
While leveraging build configurations offers flexibility and consistency in managing environment-specific settings, developers should adhere to best practices to ensure smooth deployment experiences. Some considerations include:
- Securely managing sensitive information such as API keys and credentials.
- Versioning build configurations to track changes across environments.
- Testing applications thoroughly across different environments to detect configuration-related issues early.
Conclusion:
In conclusion, build configurations play a pivotal role in managing environment-specific settings in Kotlin applications. By adopting a structured approach to defining build flavors, configuring environment-specific parameters, and automating deployment workflows, developers can ensure seamless application deployment across development, testing, and production environments.
Through effective utilization of build configurations, Kotlin developers can streamline the deployment process, minimize errors, and deliver robust applications that meet the diverse needs of end-users and stakeholders.