TECHNOLOGY
ES LINT
PRETTIER
From Messy to Meticulous: Linters & Code Formatters for JavaScript
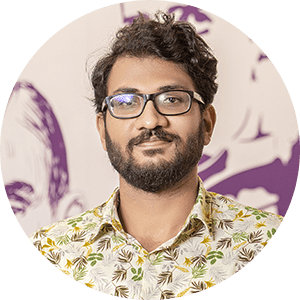
Introduction
In the dynamic world of software development, maintaining code quality and consistency is paramount. Linters and formatters play pivotal roles in ensuring codebases remain clean, readable, and error-free.
In this document, we’ll dive into discussing linters and code formatters, exploring their functionalities, benefits, and practical applications when you’re working on a React application. We will uncover how these tools streamline the development process, enhance collaboration among team members, and ultimately contribute to the creation of robust and maintainable software solutions.
Whether you’re a seasoned developer looking to optimize your workflow or a newcomer eager to learn about best practices in code quality management, this document aims to provide valuable insights and practical guidance.
Linters
What are Linters?
Linters are code analyzers that statically analyze the code for identifying and reporting issues that can lead to bugs or inconsistencies of code health and style.
Linters can help you with,
- Flagging bugs in your code from syntax errors.
- Giving you warnings when code may not be intuitive.
- Providing suggestions for common best practices.
- Keeping track of TODO’s and FIXME’s.
- Keeping a consistent code style.
ESLint
ESLint is a linter for JavaScript which can be easily integrated with some of the most commonly used IDEs and CI/CD pipelines.
This tool is completely customizable i.e., you can decide which rules to activate and which to deactivate. You can also add community plugins, configurations, and parsers to extend the functionality of ESLint.
Before we dive further into ESLint, there are some technical terms we should be familiar with.
- Plugin — A single rule in ESLint is known as a plugin.
- Config — A set of plugins that are closely related to each other i.e., a bulk of plugins.
Integrating ESLint in IDEs
Since most of us are using VS Code when working with React, let’s see how we can integrate ESLint with VS Code.
Integration is as easy as installing the ESLint extension provided Microsoft in Visual Studio Marketplace.
Integrating ESLint with Your Project
Once you have integrated ESLint with your IDE, you should install ESLint into your project. Although you can install ESLint globally on your computer, we recommend you to install it inside the project, because you can customize it project-wise.
After installation you can create the .eslintrc config file to specify the rules you want enforce.
A comprehensive guide to installing ESLint is provided in the official docs.
Common ESLint Rules
These are some of the plugins and configs we generally use to maintain the quality of the codebase.
- eslint-plugin-next - Base configuration that makes it possible to catch common issues and problems in a Next.js application. You can read the full set of rules in Configuring: ESLint.
- eslint-plugin-next/core-web-vitals - Enables rule set related to Core Web Vitals, which is helpful if you want to increase the Core Web Vitals score and SEO your application. Read more about this ruleset on Configuring: ESLint. eslint:recommended - Recommended rules by ESLint. For more details, read Rules Reference — ESLint — Pluggable JavaScript Linter.
- airbnb-typescript - Adds Airbnb typescript rules to the project. Available on npm: eslint-config-airbnb-typescript.
- eslint-plugin-import - Enables rules related to imports and exports. Read more about these rules on npm: eslint-plugin-import.
- eslint-plugin-simple-import-sort - When enabled, enforces imports to be sorted. On npm: eslint-plugin-simple-import-sort you can get more info on this plugin.
- eslint-plugin-jsx-a11y - Used for identifying the issues that are related to accessibility. Accessibility is important if you are concerned about SEO of your web application. Supported rules of this plugin and more details are available on npm: eslint-plugin-jsx-a11y.
- eslint-plugin-prettier - Allows you to run Prettier, the code formatter that we’ll be discussing, as linter rules. Read npm: eslint-plugin-prettier to understand installing and provided features of this plugin.
Remember that enabled linting rules can be changed based on your project.
Code Formatters
What are Code Formatters?
Code formatters allow you to keep a consistent code styling across the project, which will lead to increased code quality of your codebase. Code formatter will automate the processing of checking for code formatting issues and fixing them, which will allow you to focus on more important tasks, like writing the code.
Prettier
Prettier is an opinionated (has a default set of formatting rules) code formatter that supports JavaScript, TypeScript, CSS, JSX, Angular and many more.
We are using Prettier to keep a consistent code formatting across the project.
Integrating Prettier in IDEs
We can easily install the Prettier extension in the Visual Studio Marketplace to use the code formatter in VS Code.
Integrating Prettier in your project
Integrating with ESLint
Prettier can be integrated with ESLint to run styling rules as linting rules. However, some rules in ESLint might conflict with rules provided in Prettier plugin. You can config your eslintrc config file to resolve these conflicts and customize according to your needs. Follow Integrating with Linters · Prettier to get a guide on how to integrate Prettier with linters.
Integrating with Git and CI/CD Pipeline
You can use Prettier with a pre-commit tool. This can re-format your files that are marked as “staged” via git add before you commit. You can use Husky and Lint-staged to set Prettier to check formatting as a pre-commit tool. Read Pre-commit Hook · Prettier to get more info. You can refer GitHub — typicode/husky: Git hooks made easy 🐶 woof! and GitHub — lint-staged/lint-staged: 🚫💩 — Run linters on git staged files to learn more about pre-commit tools.
ESLint vs. Prettier
You might think ESLint (and pretty much all linters) and Prettier (and other code formatters) has similar responsibilities, and a bit confused about their differences. The below table will help you clearly understand the difference between these two tools.
I hope this document has laid a solid foundation for your journey in learning about Linters and Code formatters. Remember, learning is a continuous process, and mastering these tools will undoubtedly enhance your skills as a developer.
Let linting be your guide through the labyrinth of code, and formatting your brush for crafting elegance. Happy coding! 🚀🖥️